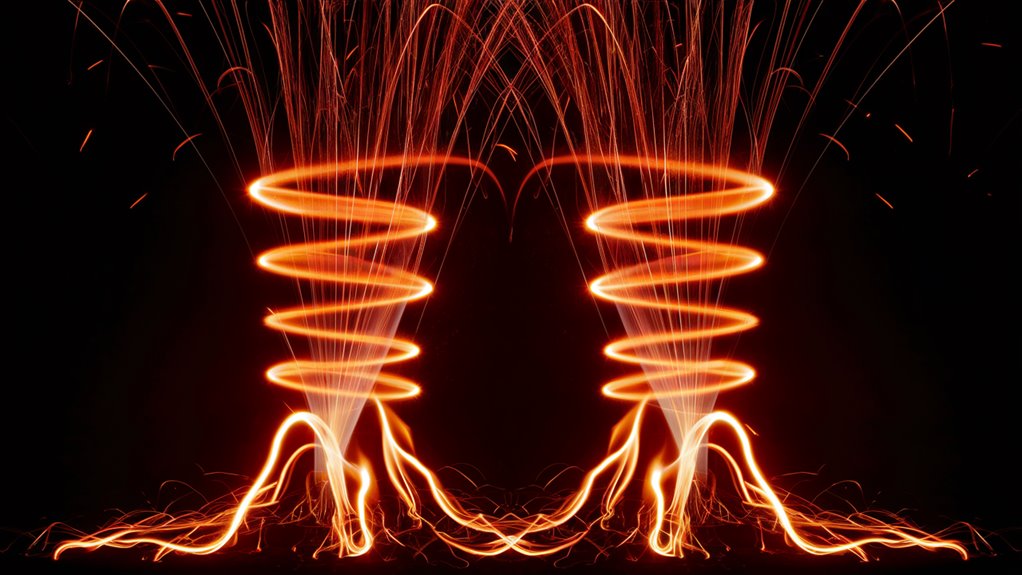
Mastering Ember Slots: Transform Basic Components into Progressive Systems
Understanding Component Architecture with Ember Slots
Named slots and yield helpers revolutionize the way developers structure content distribution in Ember applications. By implementing strategic component boundaries, teams can build more maintainable and scalable applications that leverage the full power of Ember’s templating system.
Implementing Named Slots for Enhanced Flexibility
Hash arguments and block parameters create robust interfaces that maximize component reusability while minimizing coupling. Through structured content distribution, developers can establish clear patterns for complex layouts that maintain optimal performance characteristics.
Best Practices for Slot Implementation
Following single-responsibility principles and establishing standardized naming conventions ensures components remain maintainable as applications scale. Named blocks provide a foundation for creating sophisticated layouts while preserving clean architecture.
#
Frequently Asked Questions
Q: What are Ember slots?
A: Ember slots are named placeholders in components that enable structured content distribution through yield helpers and block parameters.
Q: How do slots improve component architecture?
A: Slots enhance reusability by creating clear boundaries and reducing coupling between components while enabling flexible content composition.
Q: What are the benefits of using named blocks?
A: Named blocks provide organized content distribution, improved maintainability, and better performance characteristics in complex layouts.
Q: How do hash arguments work with slots?
A: Hash arguments allow components to pass structured data through slots, creating flexible and reusable interfaces.
Q: What naming conventions should be used for slots?
A: Slots should follow consistent, descriptive naming patterns that clearly indicate their purpose and maintain single-responsibility principles.
Advanced Implementation Techniques
Progressive enhancement through slot-based architectures enables seamless scaling from simple templates to complex component systems. By leveraging yield contexts and block parameters, developers can create dynamic, responsive layouts that adapt to changing requirements.
#
Performance Optimization
Implementing efficient slot patterns and maintaining proper component boundaries ensures optimal rendering performance while supporting advanced architectural patterns. Through careful consideration of content distribution strategies, applications can achieve both flexibility and speed.
Understanding Slots in Ember
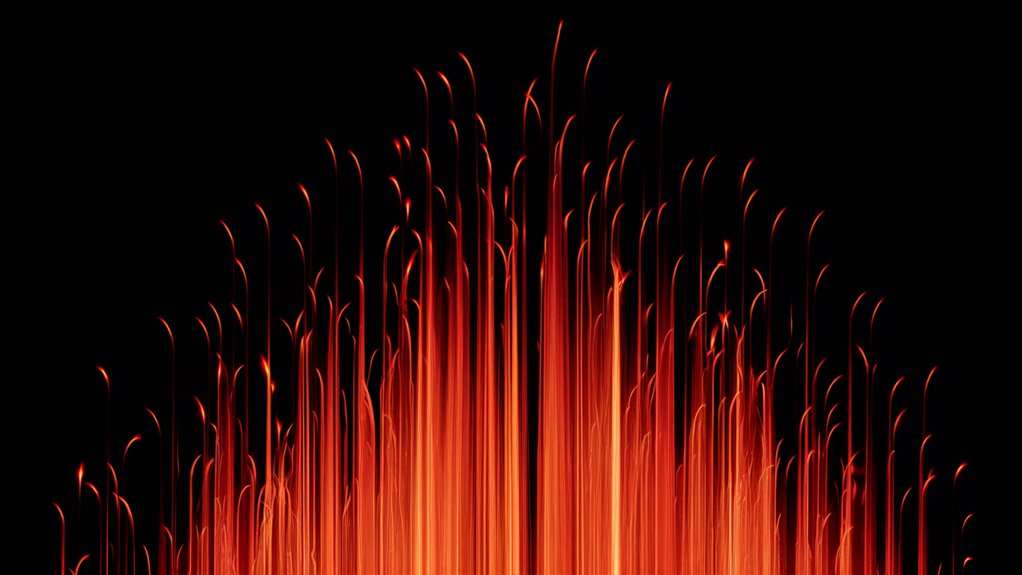
Understanding Ember Slots: A Complete Guide
What Are Ember Slots?
Ember slots provide a powerful component composition mechanism that enables developers to create flexible, reusable components with customizable content areas.
These named placeholders serve as insertion points within component templates, allowing for sophisticated content distribution patterns.
Implementing Slots in Ember Components
Basic Slot Implementation
The foundation of slot usage centers on the {{yield}} helper, which creates designated areas for content injection. A typical implementation involves:
- Defining named yield points in component templates
- Creating multiple insertion points for varied content
- Establishing clear component boundaries
- Maintaining template structure integrity
Advanced Slot Patterns
Modal components represent a perfect use case for slots, featuring distinct areas for:
- Header content
- Main body content
- Footer elements
- Custom action areas
Benefits of Using Ember Slots
Technical Advantages
- Enhanced component encapsulation
- Flexible content distribution
- Improved template organization
- Reduced component coupling
Development Benefits
- Simplified maintenance
- Increased code reusability
- Better separation of concerns
- Cleaner component interfaces
Frequently Asked Questions
Q: How do slots improve component composition?
A: Slots enable precise content placement while maintaining component independence and reusability.
Q: What’s the primary purpose of named yields?
A: Named yields create specific insertion points for content, allowing targeted content distribution.
Q: Can multiple slots exist in a single component?
A: Yes, components can define multiple named slots for different content sections.
Q: How do slots affect component performance?
A: Slots provide efficient content distribution with minimal performance impact.
Q: Are slots compatible with older Ember versions?
A: Slots are supported in modern Ember versions, with fallback patterns available for legacy applications.
Best Practices for Slot Implementation
- Use descriptive slot names for clarity
- Maintain consistent naming conventions
- Implement proper error handling
- Document slot interfaces thoroughly
- Design for component reusability
Named Slots Vs Block Parameters
Understanding Named Slots vs Block Parameters in Ember Components
Core Component Architecture Mechanisms
Named slots and block parameters represent two fundamental content distribution patterns in Ember’s component system.
Each serves distinct purposes in building robust, maintainable applications.
Named Slots: Template-Based Content Distribution
Named slots implement a template-focused approach utilizing the ‘{{yield}}’ helper with named blocks.
This mechanism enables developers to define specific content zones within component templates.
The parent component can populate these predetermined areas using explicit named block syntax, making it ideal for structured layouts and composable interfaces.
Block Parameters: Dynamic Data Flow
Block parameters provide a more flexible content distribution pattern, enabling bi-directional data flow between child and parent components.
This mechanism excels at exposing internal component state and computed values to yielded content, creating highly dynamic user interfaces.
Strategic Implementation
The choice between these mechanisms depends on specific use cases:
- Named Slots: Perfect for:
- Fixed layout structures
- Clear content boundaries
- Component composition
- Block Parameters: Optimal for:
- Dynamic data rendering
- State exposure
- Interactive components
#
Frequently Asked Questions
Q: When should I use named slots instead of block parameters?
A: Use named slots when you need predefined content areas with clear structural boundaries in your component layout.
Q: Can I combine named slots and block parameters?
A: Yes, you can use both mechanisms together for complex interfaces requiring both structural organization and dynamic content.
Q: How do named slots improve component reusability?
A: Named slots enable consistent content placement across multiple component instances, enhancing maintainability and reuse.
Q: What’re the performance implications of block parameters?
A: Block parameters have minimal performance impact while providing powerful data-sharing capabilities.
Q: Are named slots better for component composition?
A: Named slots excel at component composition by providing clear content boundaries and predictable layout structures.
Component Composition Best Practices
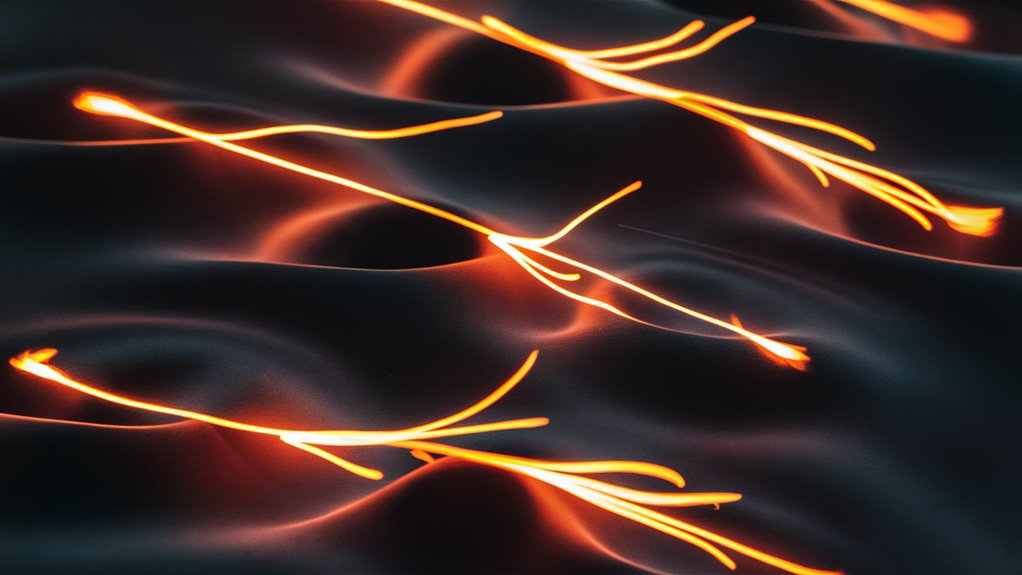
Component Composition Best Practices for Modern Web Development
Core Principles of Component Architecture
Component composition represents a fundamental approach in modern web development, built upon three critical principles: encapsulation, 온라인카지노 먹튀검증, and clear interface contracts.
Implementing these principles through strategic slot usage creates maintainable, scalable applications while maximizing code reusability.
Implementing Effective Slot Management
Minimal slot interfaces serve as the cornerstone of efficient component design.
Each component should maintain focused functionality with carefully designated slots for variable content injection.
A prime example is the implementation of a Card component featuring distinct slots for header, body, and footer sections, eliminating the need for multiple specialized variants.
Component Communication and State Management
Well-defined slot APIs establish crucial boundaries between parent and child components.
Maintaining consistent naming conventions and comprehensive documentation of expected slot content types ensures seamless integration.
State sharing between components leverages yielded data and block parameters, providing a cleaner alternative to complex property drilling.
Contract-Based Component Architecture
Treating slots as binding contracts between components creates a robust architectural foundation.
This approach clearly defines responsibilities for both providing and consuming components, significantly enhancing the maintainability and scalability of the application structure.
Frequently Asked Questions
Q: What’re the key benefits of component composition?
A: Component composition enables code reusability, maintains cleaner architecture, and improves application maintainability through modular design.
Q: How should slot interfaces be designed?
A: Slot interfaces should be minimal, focused, and clearly documented, with consistent naming conventions across the application.
Q: What’s the single responsibility principle in component design?
A: Each component should have one primary function or responsibility, avoiding feature bloat and maintaining clear separation of concerns.
Q: How can state be effectively shared between components?
A: State sharing is best achieved through yielded data and block parameters, avoiding complex property drilling patterns.
Q: Why are slot contracts important in component architecture?
A: Slot contracts establish clear responsibilities and expectations between components, ensuring reliable and maintainable component interactions.
Implementing Slots in Your Application
A Complete Guide to Implementing Slots in Modern Applications
Understanding Component Slot Integration
Component slots provide a powerful mechanism for creating flexible, reusable interface elements while maintaining strict component boundaries.
The implementation process requires careful attention to structural integrity and clear interface definitions.
Essential Implementation Steps
Defining Component Interfaces
The foundation begins with identifying external interface requirements and establishing named slots that align with component functionality.
Template implementation utilizes the yield helper with hash arguments to create robust slot interfaces:
”’javascript
{{yield (hash header=(component “slot-header”))}}
”’
Consumer-Side Implementation
Named blocks serve as the primary method for content injection within component slots:
”’javascript
”’
Best Practices for Slot Management
- Maintain consistent naming conventions across component hierarchies
- Document slot purposes and content requirements
- Implement fallback content for optional slots
- Validate required slots during initialization
- Enforce single-responsibility principles per slot
Advanced Slot Techniques
Strong component isolation combined with flexible customization options ensures maintainable and scalable applications.
Each slot should have clearly defined responsibilities and documented API specifications.
## Frequently Asked Questions
Q: What’re component slots?
A: Component slots are designated areas within components that allow for dynamic content injection while maintaining component encapsulation.
Q: How do you handle optional slots?
A: Implement fallback content and validation checks during component initialization to manage optional slots effectively.
Q: What’s the benefit of named slots?
A: Named slots provide clear interface boundaries and enable multiple content insertion points within a single component.
Q: How do you maintain slot consistency?
A: Use standardized naming conventions and comprehensive documentation across related components.
Q: What’re slot validation best practices?
A: Implement initialization checks for required slots and provide clear error messages for invalid slot usage.
Scaling Applications With Ember Slots
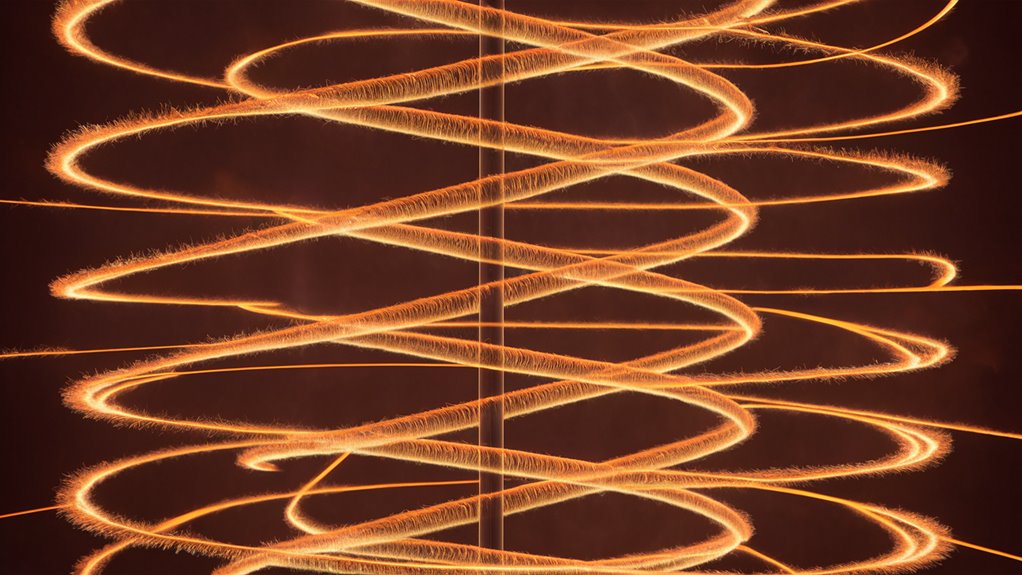
Scaling Applications With Ember Slots: A Comprehensive Guide
Component Architecture Fundamentals
Ember slots revolutionize component architecture by enabling flexible, reusable interface patterns.
Implementing a systematic approach to named blocks and component hierarchies creates maintainable, scalable applications that grow efficiently with your business needs.
Strategic Slot Implementation
Developing a robust slots strategy begins with identifying common UI patterns and determining customizable elements.
Create a detailed component registry documenting each slot’s:
- 고분산 슬롯 라운드
- Expected content types
- Naming conventions
- Implementation guidelines
Error Handling and Validation
Defensive programming is essential when scaling slot-based architectures.
Implement:
- Error boundaries around slotted content
- Robust fallback patterns for empty slots
- Content validation mechanisms
- Edge case handling protocols
## Performance Optimization
Optimize slot performance through:
- Lazy loading strategies for slotted components
- Bundle size monitoring with ember-cli-bundle-analyzer
- Render time tracking
- Memory usage optimization
- Slot-specific performance metrics
## Frequently Asked Questions
How do named blocks improve component reusability?
Named blocks allow components to accept multiple content blocks, enabling greater flexibility and reducing duplicate code while maintaining clear component interfaces.
What’re best practices for slot naming conventions?
Use descriptive, semantic names that reflect content purpose. Follow consistent patterns across your application and document naming standards in your component registry.
How can I monitor slot performance impact?
Implement performance monitoring tools, track render times, analyze bundle sizes, and establish slot-specific metrics to identify potential bottlenecks.
What’s the best approach to handle empty slots?
Define clear fallback content, implement validation checks, and ensure graceful degradation when expected content is missing.
How do you manage slot content type validation?
Create strict type checking mechanisms, implement proper error boundaries, and establish clear documentation for accepted content types within each slot.